Laravel socialite login with facebook tutorial. Here, you will learn how to implement facebook login with laravel app using the socialite package. This tutorial shows you step by step how you add the facebook login button in your laravel application.
As well as learn how to add the facebook social login button in your laravel project blade views file. And how-to simple authenticate (log in) users using socialite facebook login in laravel app.
This laravel facebook login example tutorial will guide you step by step on how to integrate Facebook social login in laravel 7, 6 version.
Laravel Facebook Login Example
Follow the below steps and implement socialite facebook login in laravel 7.x and 6.x version:
- Step 1: Install Laravel New App
- Step 2: Connect App to Database
- Step 3: Download Socialite Package
- Step 4: Create Facebook App
- Step 5: Create Auth Scaffolding
- Step 6: Add Route
- Step 7: Create Controller By Artisan Command
- Step 8: Add Facebook Login Button On Blade View
- Step 9: Run Development Server
Step 1: Install Laravel New App
Now, open your terminal and run the following command to download the latest laravel fresh setup to create laravel Facebook login app:
composer create-project --prefer-dist laravel/laravel blog
Step 2: Connect App to Database
In this step, navigate to downloaded project root directory and open .env file. Then add database details to connect your app with laravel socialite facebook login app:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=here your database name here
DB_USERNAME=here database username here
DB_PASSWORD=here database password here
Step 3: Download Socialite Package
In this step, you need to install the socialite package for facebook login. You can use the below command and install this laravel socialite package :
composer require laravel/socialite
After successfully install socialite package. You need to configure the aliases and provider in app.php file. So navigate to config directory and open app.php and add the following:
'providers' => [
// Other service providers…
Laravel\Socialite\SocialiteServiceProvider::class,
],
'aliases' => [
// Other aliases…
'Socialite' => Laravel\Socialite\Facades\Socialite::class,
],
Step 4: Create Facebook App
Now, you need to create facebook app to get the CLIENT ID and CLIENT SECRET from facebook app. To add social facebook login button in the laravel based project.
Visit the following url https://developers.facebook.com/apps/ and create a new facebook app.
1: Create facebook app with email and app name look like below picture:
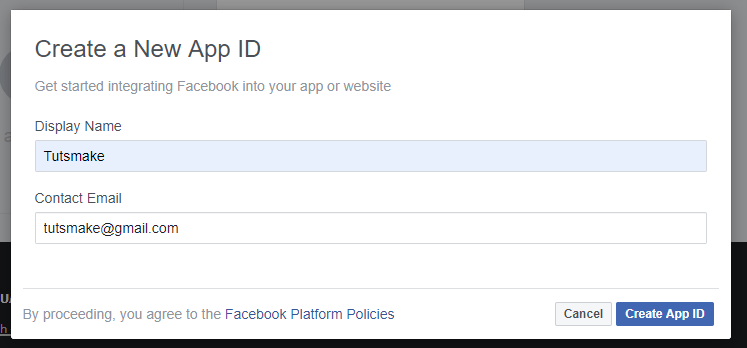
2: Then, Navigate to setting->advanced and add redirect URL, looks like below picture:

3: Now, add valid auth redirect url. So, click facebook login->setting and add valid auth redirect URL, looks like below picture:

4: Finally, Navigate to facebook developers dashboard and copy the following App ID and App SECRET, looks like below picture:
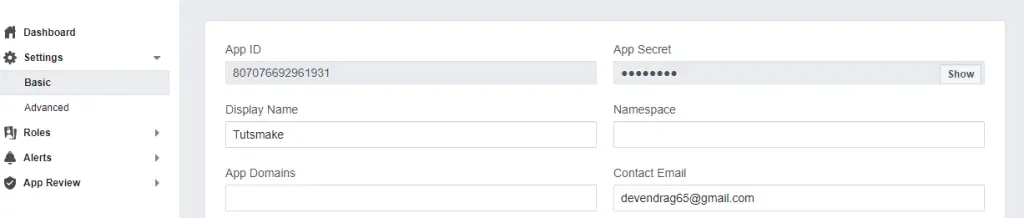
Now, Navigate to the config directory of your laravel facebook login app. And open service.php file. Then add the client id and secret id details got from facebook app in service.php file:
'facebook' => [
'client_id' => 'xxxx',
'client_secret' => 'xxx',
'redirect' => 'https://www.tutsmake.com/laravel-example/callback/facebook',
],
Next, Navigate to User.php model and add fillable property like below:
protected $fillable = [
'name', 'email', 'password', 'provider', 'provider_id'
];
Now, navigate to database/migrations directory and open create_users_table.php. Then add the following code into it:
<?php
use Illuminate\Support\Facades\Schema;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class CreateUsersTable extends Migration
{
/**
Run the migrations.
*
@return void
*/
public function up()
{
Schema::create('users', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('email')->unique()->nullable();
$table->string('provider');
$table->string('provider_id');
$table->timestamp('email_verified_at')->nullable();
$table->string('password')->nullable();
$table->rememberToken()->nullable();
$table->timestamps();
});
}
/**
Reverse the migrations.
*
@return void
*/
public function down()
{
Schema::dropIfExists('users');
}
}
Before you run PHP artisan migrate command. Navigate to app/providers/ directory and open AppServiceProvider.php. Then add the following code into it:
…
use Illuminate\Support\Facades\Schema;
….
function boot()
{
Schema::defaultStringLength(191);
}
…
Now, run the command to migrate the table:
php artisan migrate
Step 5: Create Auth Scaffolding
In this step, you need to run the following commands to create or generate auth scaffolding files. So run the following command one by one:
Install Laravel UI
composer require laravel/ui
Create Auth
php artisan ui bootstrap --auth
NPM Install
npm install
Step 6: Add Route
In this step, you need to add the following routes in the web.php file. So navigate to routes directory and open web.php file. Then add the following routes:
Route::get('/auth/redirect/{provider}', 'SocialController@redirect');
Route::get('/callback/{provider}', 'SocialController@callback');
Step 7: Create Controller By Artisan Command
Now, run the following command to create a controller name SocialController.php file:
php artisan make:controller SocialController
Then navigate to app/http/controllers/ directory and open SocialController.php. Then add the following code into your SocialController.php file:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Validator,Redirect,Response,File; use Socialite; use App\User; class SocialController extends Controller { public function redirect($provider) { return Socialite::driver($provider)->redirect(); } public function callback($provider) { $getInfo = Socialite::driver($provider)->user(); $user = $this->createUser($getInfo,$provider); auth()->login($user); return redirect()->to('/home'); } function createUser($getInfo,$provider){ $user = User::where('provider_id', $getInfo->id)->first(); if (!$user) { $user = User::create([ 'name' => $getInfo->name, 'email' => $getInfo->email, 'provider' => $provider, 'provider_id' => $getInfo->id ]); } return $user; } }
Step 8: Add Facebook Login Button On Blade View
In this step, you need to add Facebook login button in blade views file.
So navigate to resources/views/Auth directory and open login.blade.php. Then add Facebook social login button in login.blade.php file:
<hr> <div class="form-group row mb-0"> <div class="col-md-8 offset-md-4"> <a href="{{ url('/auth/redirect/facebook') }}" class="btn btn-primary"><i class="fa fa-facebook"></i> Facebook</a> </div> </div>
Now, navigate to resources/views/Auth directory and open register.blade.php. Then add Facebook social login button in register.blade.php file:
<hr> <div class="form-group row mb-0"> <div class="col-md-8 offset-md-4"> <a href="{{ url('/auth/redirect/facebook') }}" class="btn btn-primary"><i class="fa fa-facebook"></i> Facebook</a> </div> </div>
Step 9: Run Development Server
Now, you need to start the development server using PHP artisan run command to start your laravel facebook login app:
php artisan serve
Finally, you are ready to run our facebook login laravel examples.
Open your browser and hit the following URL into it:
http://127.0.0.1:8000/login Or direct hit in your browser http://localhost/blog/public/login
Conclusion
In this laravel facebook social login tutorial, You have successfully added a login button on our laravel based project and using the facebook button. you have also implemented register code in this example and learn how to users log in with facebook in Laravel.
Recommended Laravel Posts
- Laravel Twitter Login Example Using Socialite Package
- Laravel Linkedin Login Tutorial With Live Demo
- Github Login in laravel 6 with Example
- Laravel Socialite Google Login Example
- Laravel Multi Auth( Authentication) Example Tutorial
- Laravel Custom Login Registration Example Tutorial
- Laravel REST API (Login & Registration) Authentication
If you have any questions or thoughts to share, use the comment form below to reach us.