If you want to upload multipart/form-data file without refreshing and reloading the page using jQuery and Ajax. then this tutorial is for you.
While jQuery Ajax file upload is relatively simple, adding multipart/form-data with validation can greatly improve the user experience and overall robustness of the application. In this tutorial, you will learn step-by-step how to use jQuery Ajax to upload files with multipart/form-data validation.
How to Upload file using jQuery AJAX with Validation Tutorial
By using the following steps, you can post/send or upload file with multipart/form-data and validation with jQuery Ajax:
- Step 1 – Create HTML Form with File upload Field
- Step 2 – Include jQuery Library in HTML
- Step 3 – Create jQuery Ajax Code to Upload File multipart/form-data
Step 1 – Create HTML Form with File upload Field
In this step, you need to create an HTML form for file uploads or FormData and an extra field.
<form id="uploadForm"> <input type="file" name="file" id="fileInput" /> <input type="submit" value="Upload File" /> </form> <div id="response"></div>
Step 2 – Include jQuery Library in HTML
To include jQuery in your HTML file, place the <script>
tag containing the CDN URL in the <head>
section or just before the closing </body>
tag. This ensures that the library is loaded before any scripts that depend on jQuery.
Here’s an example of including jQuery using the CDN:
<!DOCTYPE html> <html> <head> <title>jQuery Ajax File Upload multipart/form-data Validation - Tutsmake.com</title> <script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> </head> <body> <!-- Your HTML content here --> <script> // Your JavaScript code using jQuery here $(document).ready(function() { // jQuery code goes here }); </script> </body> </html>
Step 3 – Create jQuery Ajax Code to Upload File multipart/form-data
In this step, you need to create a jquery Ajax code for sending or uploading a file with multipart/form-data to the server.
$(document).ready(function () { $("#uploadForm").submit(function (e) { e.preventDefault(); var formData = new FormData(); formData.append("file", $("#fileInput")[0].files[0]); $.ajax({ url: "upload.php", type: "POST", data: formData, processData: false, contentType: false, success: function (response) { $("#response").html(response); }, error: function () { $("#response").html("Error occurred during the upload."); }, }); }); });
While the above code allows your users to upload files, it lacks the necessary validation checks to ensure that the submitted data is accurate and secure. Here are some validation techniques for enhancing jQuery Ajax file uploads with multipart/form-data:
- File Size Validation: Limit the file size to prevent large files from affecting server performance and taking up unnecessary storage space. You can use the
File
object’ssize
property to check the file size before uploading. - File Type Validation: Ensure that users can only upload specific file types that your application supports. Use the
File
object’stype
property to validate the file’s MIME type. - Client-Side Input Validation: Before initiating the Ajax request, perform client-side input validation to check if the user has selected a file. Additionally, you can verify other form fields to ensure they meet specific requirements.
- Server-Side Validation: Always validate files on the server-side as well. Client-side validation can be bypassed, so server-side validation is crucial for data integrity and security. Verify the file size, type, and other criteria on the server.
FAQs
- How to add extra fields or data with Form data in jQuery ajax?
- ajax FormData: Illegal invocation
- How to send multipart/FormData or files with jQuery.ajax?
See the following faqs for jQuery Ajax Form Submit;
1. How to add extra fields with Form data in jQuery ajax?
The append()
method of the FormData
interface appends a new value onto an existing key inside a FormData
object, or adds the key if it does not already exist.
// Create an FormData object var data = new FormData(form); // If you want to add an extra field for the FormData data.append("CustomField", "This is some extra data, testing");
2. ajax FormData: Illegal invocation
jQuery tries to transform your FormData object to a string, add this to your $.ajax call:
processData: false, contentType: false
3. How to send multipart/FormData or files with jQuery.ajax?
In this step, you will learn how to send multiple files using jQuery ajax. Let’s see the below code Snippet:
var data = new FormData(); jQuery.each(jQuery('#file')[0].files, function(i, file) { data.append('file-'+i, file); }); $.ajax({ url: 'upload.php', data: data, cache: false, contentType: false, processData: false, method: 'POST', success: function(data){ console.log('success'); } });
Note
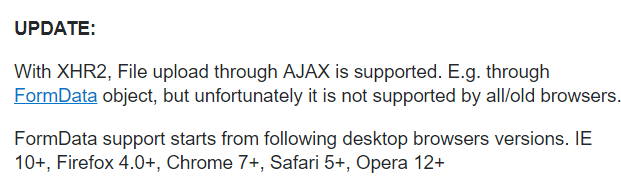
Conclusion
In this tutorial guide, you have learned how to post/send or upload file multipart/form-data with validation with jQuery and Ajax.
Recommended jQuery Tutorial
If you have any questions or thoughts to share, use the comment form below to reach us.