Laravel 8 Send email using office365 example. In this tutorial, you will learn how to send email in laravel 8 using office365. As well as learn how to integrate office365 with laravel 8 app.
Note that, you can also use several SMTP drivers details (Mailgun, Postmark, Amazon SES, and sendmail) in .env file for sending email in laravel 8.
In this tutorial will guide you step by step on sending emails in laravel 8 using office365. So, you need to follow all the below-given steps one by one. And you can send email in laravel 8.
First of all, open your office365 app and set up app passwords login to your account click Manage security and privacy.
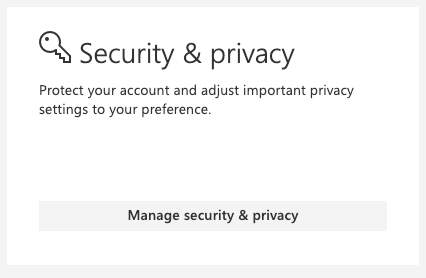
Next click on create and manage app passwords.

All your app passwords will be listed on this page, to add a new one click create.
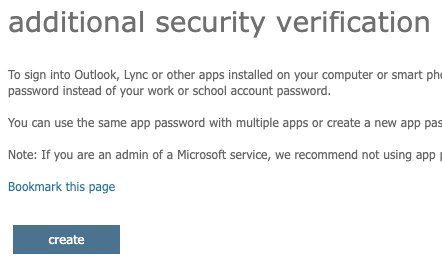
Enter a name for the app password such as your website name.
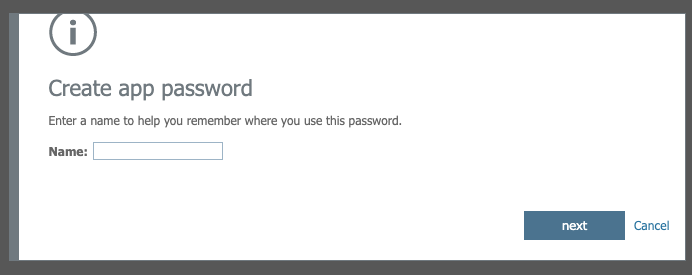
Note down the generated app password it will not be displayed again. (the below is just an example)

Now with the pass password, you’re ready to start sending emails with SMTP.
Laravel 8 Send Email Example
- Step 1 – Install Laravel 8 App
- Step 2 – Configuration SMTP in .env
- Step 3 – Create Mailable Class
- Step 4 – Add Email Send Route
- Step 5 – Create Directory And Blade View
- Step 6 – Create Email Controller
- Step 7 – Run Development Server
Step 1 – Install Laravel 8 App
In this step, use the following command to install or download laravel 8 application:
composer create-project --prefer-dist laravel/laravel blog
Step 2 – Configuration SMTP in .env
In this step, you need to configure smtp details in .env file like following:
MAIL_DRIVER=smtp MAIL_HOST=smtp.office365.com MAIL_PORT=587 MAIL_USERNAME=email MAIL_PASSWORD=app_password MAIL_ENCRYPTION=tls MAIL_FROM_ADDRESS=email MAIL_FROM_NAME="your app name"
Step 3 – Create Mailable Class
In this step, use the below given command to create NotifyMail mailable class:
php artisan make:mail NotifyMail
<?php namespace App\Mail; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Mail\Mailable; use Illuminate\Queue\SerializesModels; class NotifyMail extends Mailable { use Queueable, SerializesModels; /** * Create a new message instance. * * @return void */ public function __construct() { // } /** * Build the message. * * @return $this */ public function build() { return $this->view('view.name'); } }
By whatever name you will create an email template. That you want to send. Do not forget to add an email template name in build class of the above created notifymail class.
return $this->view('view.name'); to return $this->view('emails.demoMail');
In next step, we will create email template named demoMail.blade.php inside resources/views/emails directory. That’s why we have added view name email.
Step 4 – Add Send Email Route
In this step, open /web.php, so navigate to routes directory. And then add the following routes for send email:
use App\Http\Controllers\SendEmailController; Route::get('send-email', [SendEmailController::class, 'index']);
Step 5 – Create Directory And Blade View
In this step, create directory name emails inside resources/views directory. Then create an demoMail.blade.php blade view file inside resources/views/emails directory. And update the following code into it:
<!DOCTYPE html> <html> <head> <title>Laravel 8 Send Email Example</title> </head> <body> <h1>This is test mail from Tutsmake.com</h1> <p>Laravel 8 send email example</p> </body> </html>
Step 6 – Create Send Email Controller
In this step, use the following command to create controller name SendEmailController:
php artisan make:controller SendEmailController
Then navigate to app/Http/Controllers directory and open SendEmailController.php. Then update the following code into it:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Mail; class SendEmailController extends Controller { public function sendEmail() { Mail::to('receiver-email-id')->send(new NotifyMail()); if (Mail::failures()) { return response()->Fail('Sorry! Please try again latter'); }else{ return response()->success('Great! Successfully send in your mail'); } } }
Step 7 – Run Development Server
In this step, use this PHP artisan serve command to start your server locally:
php artisan serve
Then open browser and fire the following URL on it:
http://127.0.0.1:8000/send-email
Conclusion
Laravel 8 send an email example, you have learned how to create a mailable class in laravel 8. And using this class on how to send emails in laravel 8.
Recommended Laravel Posts
If you have any questions or thoughts to share, use the comment form below to reach us.