In this tutorial, you will learn how to make CRUD rest API in PHP Codeigniter 4 frameworks with MySQL database and use it with postman application.
How to Create RESTful APIs in CodeIgniter 4
Use the following steps to create rest API in CodeIgniter 4 framework with MySQL database and call endpoints with postman application:
- Step 1: Setup Database and Table
- Step 2: Setup Codeigniter Project
- Step 3: Basic Configurations
- Step 4: Setup Database Credentials
- Step 5: Create Model
- Step 6: Create Controller
- Step 7: Start Development server
Step 1: Setup Database and Table
First of all, create database by using the following SQL query command:
CREATE DATABASE demo;
After that, select your database and run the following sql query to create a Product table into your database:
CREATE TABLE product(
product_id INT(11) PRIMARY KEY AUTO_INCREMENT,
product_name VARCHAR(200),
product_price DOUBLE
)ENGINE=INNODB;
Step 2: Setup Codeigniter 4 Project
In this step, we will download the latest version of Codeigniter 4, Go to this link https://codeigniter.com/download Download Codeigniter 4 fresh new setup and unzip the setup in your local system xampp/htdocs/ . And change the download folder name “demo”
Step 3: Basic Configurations
Next, we will set some basic configuration on the app/config/app.php file, so let’s go to application/config/config.php and open this file on text editor.
Set Base URL like this
public $baseURL = 'http://localhost:8080'; To public $baseURL = 'http://localhost/demo/';
Step 4: Setup Database Credentials
In this step, we need to connect our project to the database. we need to go app/Config/Database.php and open database.php file in text editor. After opening the file in a text editor, We need to set up database credentials in this file like below.
public $default = [
'DSN' => '',
'hostname' => 'localhost',
'username' => 'root',
'password' => '',
'database' => 'demo',
'DBDriver' => 'MySQLi',
'DBPrefix' => '',
'pConnect' => false,
'DBDebug' => (ENVIRONMENT !== 'production'),
'cacheOn' => false,
'cacheDir' => '',
'charset' => 'utf8',
'DBCollat' => 'utf8_general_ci',
'swapPre' => '',
'encrypt' => false,
'compress' => false,
'strictOn' => false,
'failover' => [],
'port' => 3306,
];
Step 5: Create Model
So go to app/Models/ and create here one model. And you need to create one model name ProductModel.php and update the following code into your ProductModel.php file:
<?php namespace App\Models;
use CodeIgniter\Model;
class ProductModel extends Model
{
protected $table = 'product';
protected $primaryKey = 'product_id';
protected $allowedFields = ['product_name','product_price'];
}
Step 6: Create Controller
Now, Navigate app/Controllers and create a controller name Products.php. Then update the following method into Products.php controller file:
<?php namespace App\Controllers;
use CodeIgniter\RESTful\ResourceController;
use CodeIgniter\API\ResponseTrait;
use App\Models\ProductModel;
class Products extends ResourceController
{
use ResponseTrait;
// get all product
public function index()
{
$model = new ProductModel();
$data = $model->findAll();
return $this->respond($data);
}
// get single product
public function show($id = null)
{
$model = new ProductModel();
$data = $model->getWhere(['product_id' => $id])->getResult();
if($data){
return $this->respond($data);
}else{
return $this->failNotFound('No Data Found with id '.$id);
}
}
// create a product
public function create()
{
$model = new ProductModel();
$data = [
'product_name' => $this->request->getVar('product_name'),
'product_price' => $this->request->getVar('product_price')
];
$model->insert($data);
$response = [
'status' => 201,
'error' => null,
'messages' => [
'success' => 'Data Saved'
]
];
return $this->respondCreated($response);
}
// update product
public function update($id = null)
{
$model = new ProductModel();
$input = $this->request->getRawInput();
$data = [
'product_name' => $input['product_name'],
'product_price' => $input['product_price']
];
$model->update($id, $data);
$response = [
'status' => 200,
'error' => null,
'messages' => [
'success' => 'Data Updated'
]
];
return $this->respond($response);
}
// delete product
public function delete($id = null)
{
$model = new ProductModel();
$data = $model->find($id);
if($data){
$model->delete($id);
$response = [
'status' => 200,
'error' => null,
'messages' => [
'success' => 'Data Deleted'
]
];
return $this->respondDeleted($response);
}else{
return $this->failNotFound('No Data Found with id '.$id);
}
}
}
In the above controller method works as follow:
- Index() – This is used to fetch all product.
- create() – This method is used to insert product info into DB table.
- update() – This is used to validate the form data server-side and update it into the MySQL database.
- show() – This method is used to fetch single product info into DB table.
- delete() – This method is used to delete data from the MySQL database.
Now “Routes.php” file from App/Configuration folder and define route in it:
$Route->get('/', 'home :: index');
Then, change the following:
$Route->resource('product');
This configuration allows us to access the following EndPoint:
Step 7: Start Development server
Open your terminal and run the following command to start development server:
php spark serve
Next, Open the postman app to call above created APIs as follow:
1: Get all products info from DB table, you can call get all product info api in postman app as follow:
http://localhost:8080/products

2: And if you want to get single product info, you can use the as follow in postman app:
http://localhost:8080/products/10
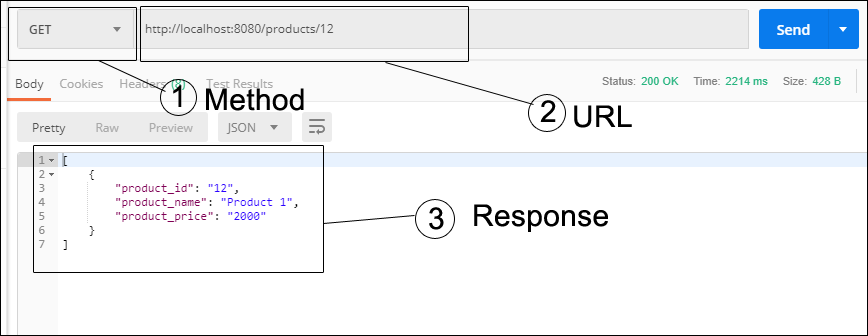
3: For insert new product info into DB table, you can call create apis as follow:
http://localhost:8080/products

4: For update info into DB table using the update api, you can call update info api as follow:

5: For delete product info using codeigniter api, you can call delete api as follow:
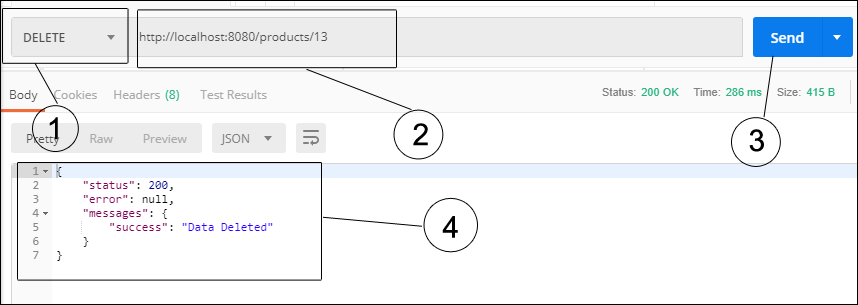
Conclusion
In this Codeigniter 4 rest API example tutorial, you have learned how to create restful API in Codeigniter 4 framework.
Recommended Codeigniter Posts
If you have any questions or thoughts to share, use the comment form below to reach us.
I love your tutorial. Thanks so much. Can i request? How about tutorial for Flutter + Restful API? CRUD. Thanks so much. I also interested with your work. Please leave me a reply and your contact number.