Laravel 8 store backup on google drive example. In this tutorial, you will learn how to store backup on google drive using google drive APIs in laravel 8 apps.
Google Drive integration is easy in Laravel 8 app. And this is because of the spatie/laravel-backup package.
Laravel 8 Backup Store On Google Drive
Use the following steps to store the backup on google drive in laravel 8 apps; as follows:
- Step 1 – Setup Google Drive Account
- Step 2 – Install Laravel 8 App
- Step 3 – Connecting App to Database
- Step 4 – Install spatie/laravel-backup
- Step 5 – Setup Google Drive as Filesystem in Laravel
- Step 6 – Configure Google Drive Details
- Step 7 – Execute Backup Command
Step 1 – Setup Google Drive Account
Make sure this before integrating Google Drive in laravel 8. that you have google client id and secret id. And if not, then you can get by following the steps given below. Otherwise, skip the get google key id steps and follow google integration in laravel 8 app:
You can create Google App in Google Developer Console by following the steps given below.
Step 1 – Visit Google Developer Console. And create a new project as following in below picture:

Step 2 – At that point visit to “Library” of google search console and search for “Google Drive API“:

Step 3 – Then enable the Google Drive API.

Step 4 – Then, Visit “Credentials” and click on the tab “OAuth Consent Screen“. Here you will get options to select user type, select “External” option, and click the “CREATE” button.

Step 5 – Now fill the form with following details like “App Name“, “User support email” and “Email” in the Developer contact information and click Save And Continue button. For other forms “Scopes“, “Test Users” and “Summary” click on Save And Continue button and go to the dashboard. Don’t worry about the other fields.

Step 6 – On the OAuth consent screen, you will see that the App’s Publishing status is testing. Here we need to publish the App. So, click the Publish App button and confirm.
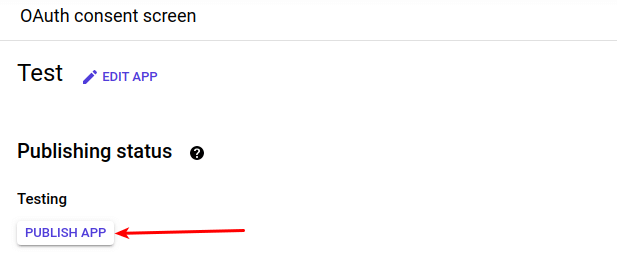
Step 7 – Now, Visit back to Credentials, click the button that says “Create Credentials” and select “OAuth Client ID“.
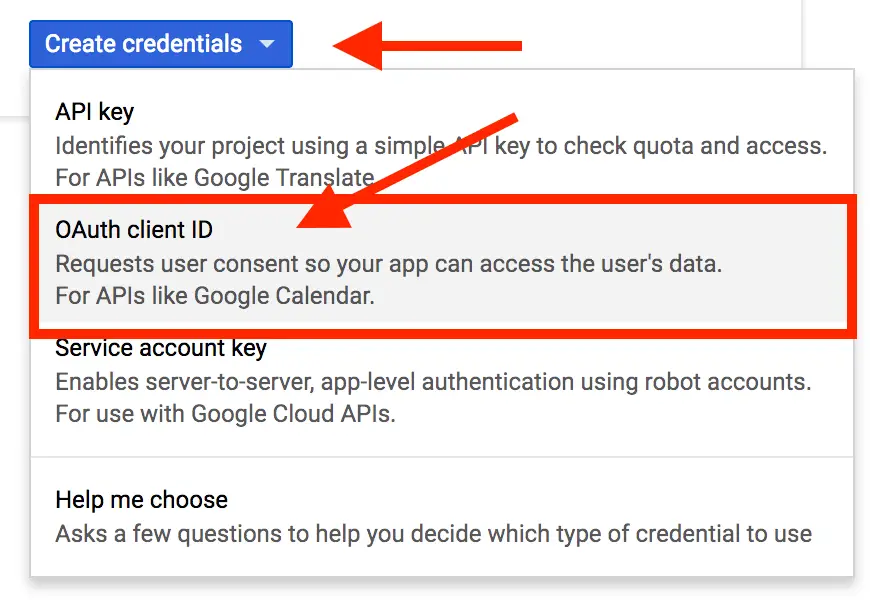
Step 8 – For “Application type” choose “Web Application” and give it a name.
Enter your “Authorized redirect URIs”, your production URL (https://tutsmake.com) — or create a separate production key later
Also add https://developers.google.com/oauthplayground temporarily, because you will need to use that in the next step.

Finally, Click Create and take note of your Client ID and Client Secret.
Step 9 – Now head to https://developers.google.com/oauthplayground.
In the top right corner, click the settings icon, check “Use your own OAuth credentials” and paste your Client ID and Client Secret.
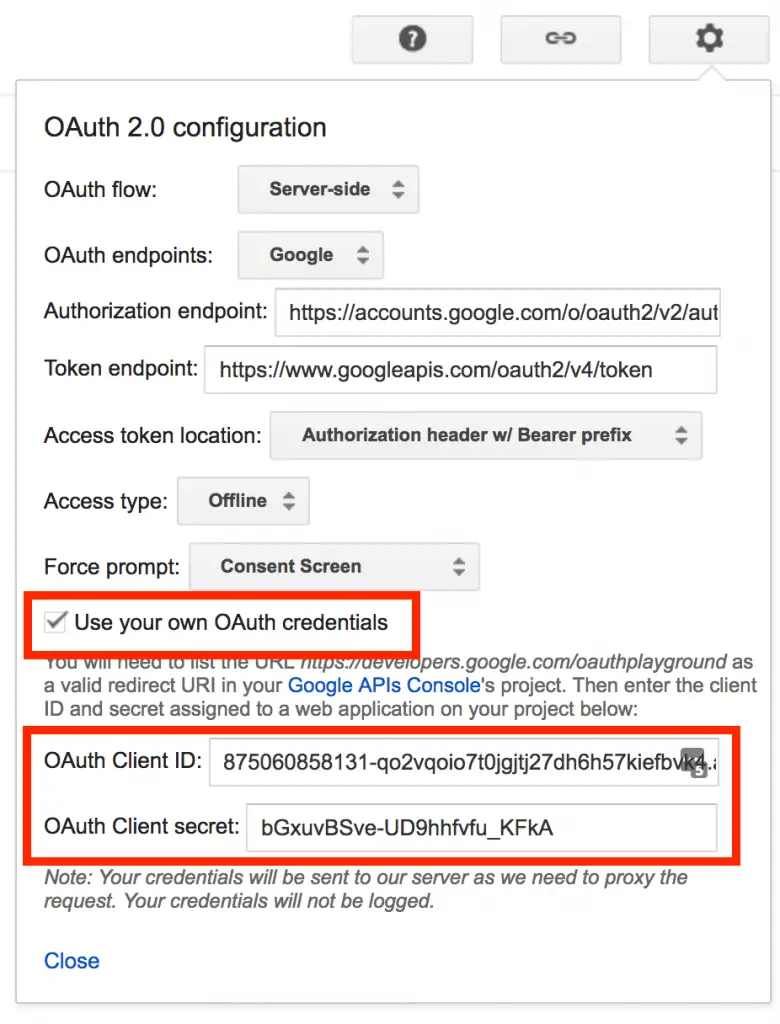
Step 10 – on the left, scroll to “Drive API v3”, expand it, and check each of the scopes.
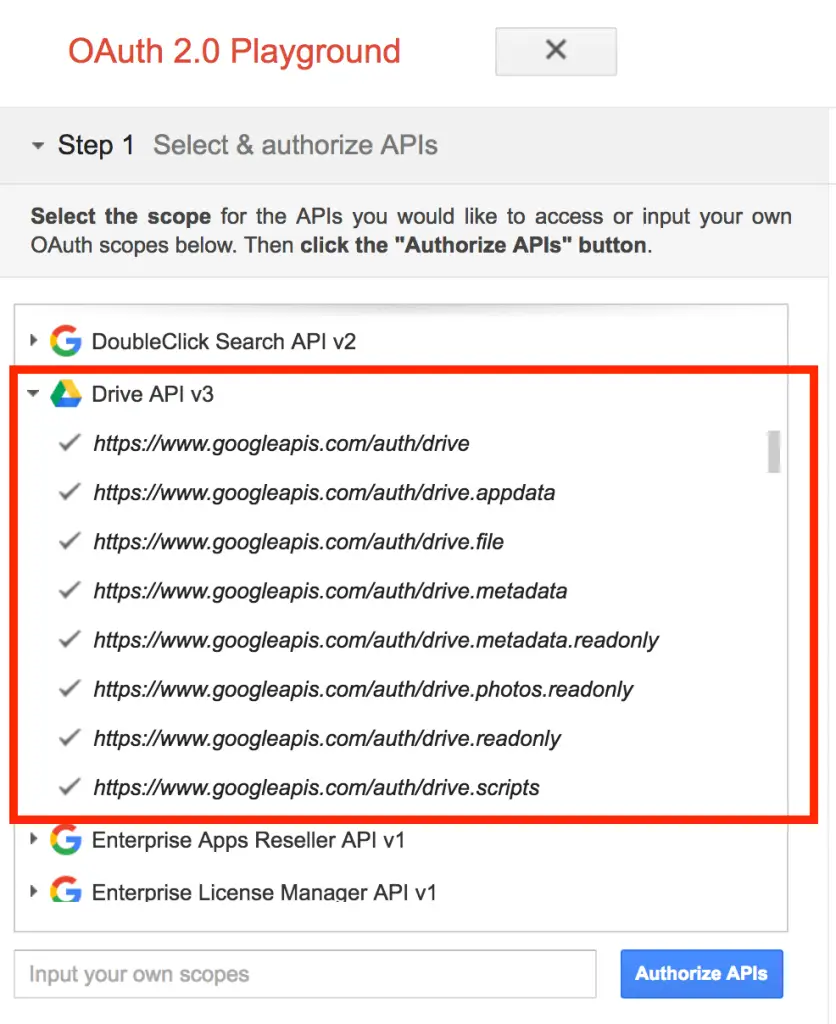
Click “Authorize APIs” and allow access to your account when prompted.
Step 11 – When you get to step 10, check “Auto-refresh the token before it expires” and click “Exchange authorization code for tokens“.
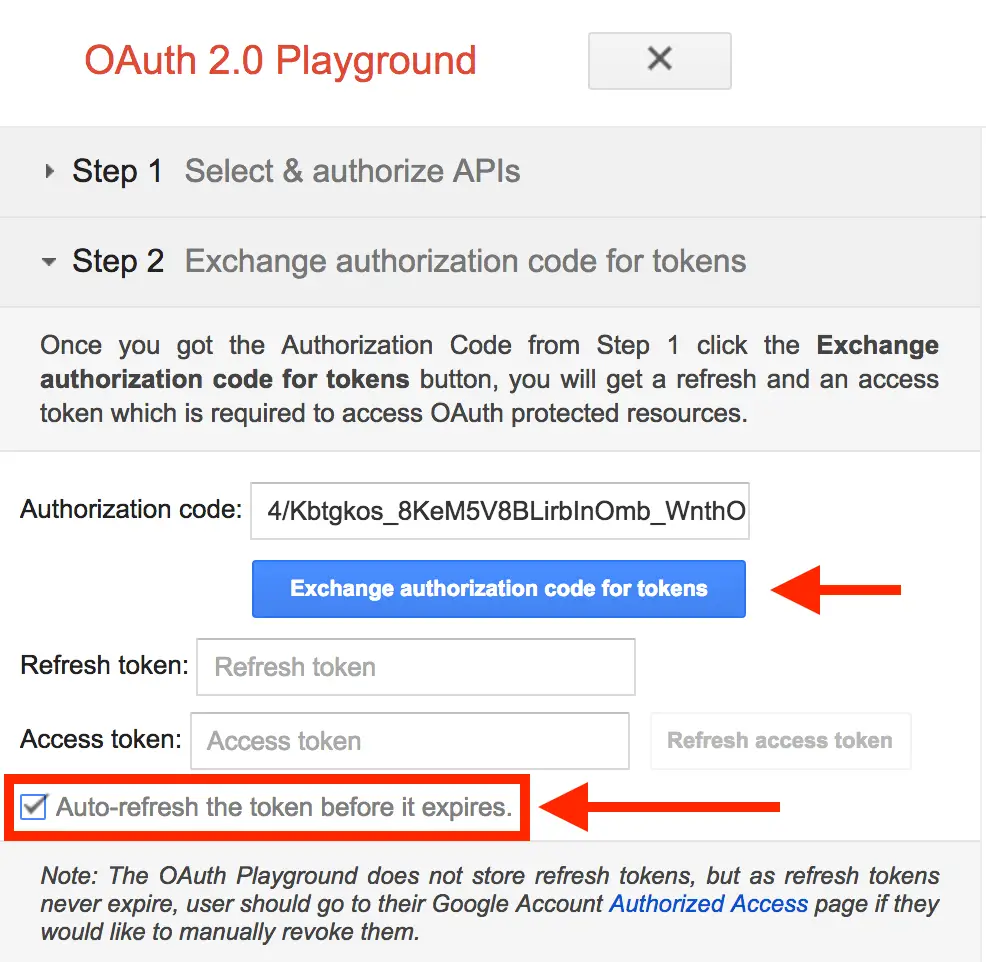
Step 12 – Now, complete this step and click on step 1 again and you should see your refresh token.

Step 13 – Now you need to folder ID that is optional. If you set folder ID to null
, in that case, it is going to store backup files in the root path of Google Drive.
You can get the folder ID from the URL, as shown in the below image.

Now, you have got Google Client ID, CLIENT SECRET, REFRESH TOKEN, and FOLDER ID. Please save it in any text file. Because you need to update these values in the .env
file of your laravel 8 app.
Step 2 – Install Laravel 8 App
In this step, open your terminal and navigate to the local webserver directory. Then type the following command on the terminal to download laravel 8 app:
composer create-project --prefer-dist laravel/laravel Blog
Step 3 – Connecting App to Database
In this step, navigate to the root directory of the download laravel app. And open .env file. Then configure database details like the following:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=here your database name here
DB_USERNAME=here database username here
DB_PASSWORD=here database password here
Step 4 – Install spatie/laravel-backup
In this step, execute the following command on terminal to install spatie/laravel-backup package in laravel 8 app:
composer require spatie/laravel-backup
Then execute the following command on terminal to publish this installed package:
php artisan vendor:publish --provider="Spatie\Backup\BackupServiceProvider"
Note that, it will publish the configuration file in config/backup.php
. Now, configure your backup according to your requirement.
Now, you need to add google
in the disk option in the config/backup.php
.
'disks' => [ 'google', 'local', ],
Step 5 – Setup Google Drive as Filesystem in Laravel
In this step, you need to execute the following command on terminal to install a Filesystem adapter for Google drive. So, run the following command in your terminal:
composer require nao-pon/flysystem-google-drive:~1.1
php artisan make:provider GoogleDriveServiceProvider
Then, inside the boot()
method add the Google driver for the Laravel filesystem:
// app/Providers/GoogleDriveServiceProvider.php public function boot() { \Storage::extend('google', function ($app, $config) { $client = new \Google_Client(); $client->setClientId($config['clientId']); $client->setClientSecret($config['clientSecret']); $client->refreshToken($config['refreshToken']); $service = new \Google_Service_Drive($client); $adapter = new \Hypweb\Flysystem\GoogleDrive\GoogleDriveAdapter($service, $config['folderId']); return new \League\Flysystem\Filesystem($adapter); }); }
After this, register the service provider by adding the following line in the providers
array of config/app.php
.
'providers' => [ // ... App\Providers\GoogleDriveServiceProvider::class, ];
Step 6 – Configure Google Drive Details
In this step, configure Google drive app with this laravel app. So, open your laravel 8 project in any text editor. Then navigate the config directory and open filesystem.php file and add the client id, secret and callback url:
return [ // ... 'disks' => [ // ... 'google' => [ 'driver' => 'google', 'clientId' => env('GOOGLE_DRIVE_CLIENT_ID'), 'clientSecret' => env('GOOGLE_DRIVE_CLIENT_SECRET'), 'refreshToken' => env('GOOGLE_DRIVE_REFRESH_TOKEN'), 'folderId' => env('GOOGLE_DRIVE_FOLDER_ID'), ], // ... ], // ... ];
And also you need to update .env
file of laravel 8 app. In this environment file you need to add the following Google drive credentials:
GOOGLE_DRIVE_CLIENT_ID=xxx.apps.googleusercontent.com GOOGLE_DRIVE_CLIENT_SECRET=xxx GOOGLE_DRIVE_REFRESH_TOKEN=xxx GOOGLE_DRIVE_FOLDER_ID=null
Step 7 – Execute Backup Command
In this step, open your terminal and execute the following command to check the backup file is created or not:
php artisan backup:run
Conclusion
Laravel 8 store backup on google drive example tutorial, you have learned how to store laravel 8 app backup on google drive using spatie/
very good instructions and help novice programmers, good job thanks