Laravel 8 CRUD operation application; In this tutorial, you will learn step by step how to build simple crud operation app in laravel 8. And how to validate add & update form data on server-side in laravel 8 crud app.
CRUD Meaning: CRUD is an acronym that comes from the world of computer programming and refers to the four functions that are considered necessary to implement a persistent storage application: create, read, update and delete.
This laravel 8 crud operation step by step tutorial will implement a simple company crud operation app in laravel 8 app with validation. Using this crud app, you can learn how to insert, read, update and delete data from database in laravel 8.
Laravel 8 CRUD Tutorial by Example
- Step 1 – Download Laravel 8 App
- Step 2 – Setup Database with App
- Step 3 – Create Company Model & Migration For CRUD App
- Step 4 – Create Routes
- Step 5 – Create Company CRUD Controller By Artisan Command
- Step 6 – Create Blade Views File
- Make Directory Name Companies
- index.blade.php
- create.blade.php
- edit.blade.php
- Step 7 – Run Laravel CRUD App on Development Server
Step 1 – Download Laravel 8 App
First of all, download or install laravel 8 new setup. So, open the terminal and type the following command to install the new laravel 8 app into your machine:
composer create-project --prefer-dist laravel/laravel LaravelCRUD
Step 2 – Setup Database with App
Setup database with your downloaded/installed laravel 8 app. So, you need to find .env file and setup database details as following:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=database-name DB_USERNAME=database-user-name DB_PASSWORD=database-password
Step 3 – Create Company Model & Migration For CRUD App
Open again your command prompt. And run the following command on it. To create model and migration file for form:
php artisan make:model Company -m
After that, open create_companies_table.php file inside LaravelCRUD/database/migrations/ directory. And the update the function up() with following code:
public function up() { Schema::create('companies', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email'); $table->string('address'); $table->timestamps(); }); }
Then, open again command prompt and run the following command to create tables into database:
php artisan migrate
Step 4 – Create Routes
Then create routes for laravel crud app. So, open web.php file from the routes directory of laravel CRUD app. And update the following routes into the web.php file:
use App\Http\Controllers\CompanyCRUDController; Route::resource('companies', CompanyCRUDController::class);
Step 5 – Create Company CRUD Controller By Artisan Command
Create a controller by using the following command on the command prompt to create a controller file:
php artisan make:controller CompanyCRUDController
After that, visit at app/Http/controllers and open CompanyCRUDController.php file. And update the following code into it:
<?php namespace App\Http\Controllers; use App\Models\Company; use Illuminate\Http\Request; class CompanyCRUDController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index() { $data['companies'] = Company::orderBy('id','desc')->paginate(5); return view('companies.index', $data); } /** * Show the form for creating a new resource. * * @return \Illuminate\Http\Response */ public function create() { return view('companies.create'); } /** * Store a newly created resource in storage. * * @param \Illuminate\Http\Request $request * @return \Illuminate\Http\Response */ public function store(Request $request) { $request->validate([ 'name' => 'required', 'email' => 'required', 'address' => 'required' ]); $company = new Company; $company->name = $request->name; $company->email = $request->email; $company->address = $request->address; $company->save(); return redirect()->route('companies.index') ->with('success','Company has been created successfully.'); } /** * Display the specified resource. * * @param \App\company $company * @return \Illuminate\Http\Response */ public function show(Company $company) { return view('companies.show',compact('company')); } /** * Show the form for editing the specified resource. * * @param \App\Company $company * @return \Illuminate\Http\Response */ public function edit(Company $company) { return view('companies.edit',compact('company')); } /** * Update the specified resource in storage. * * @param \Illuminate\Http\Request $request * @param \App\company $company * @return \Illuminate\Http\Response */ public function update(Request $request, $id) { $request->validate([ 'name' => 'required', 'email' => 'required', 'address' => 'required', ]); $company = Company::find($id); $company->name = $request->name; $company->email = $request->email; $company->address = $request->address; $company->save(); return redirect()->route('companies.index') ->with('success','Company Has Been updated successfully'); } /** * Remove the specified resource from storage. * * @param \App\Company $company * @return \Illuminate\Http\Response */ public function destroy(Company $company) { $company->delete(); return redirect()->route('companies.index') ->with('success','Company has been deleted successfully'); } }
Step 6 – Create Blade Views File
Create the directory and some blade view, see the following:
- Make Directory Name Companies
- index.blade.php
- create.blade.php
- edit.blade.php
Create directory name companies inside resources/views directory.
Next, you need to create index.blade.php, create.blade.php and edit.blade inside the companies directory.
Add the following code in index.blade.php:
index.blade.php:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Laravel 8 CRUD Tutorial From Scratch</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" > </head> <body> <div class="container mt-2"> <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2>Laravel 8 CRUD Example Tutorial</h2> </div> <div class="pull-right mb-2"> <a class="btn btn-success" href="{{ route('companies.create') }}"> Create Company</a> </div> </div> </div> @if ($message = Session::get('success')) <div class="alert alert-success"> <p>{{ $message }}</p> </div> @endif <table class="table table-bordered"> <tr> <th>S.No</th> <th>Company Name</th> <th>Company Email</th> <th>Company Address</th> <th width="280px">Action</th> </tr> @foreach ($companies as $company) <tr> <td>{{ $company->id }}</td> <td>{{ $company->name }}</td> <td>{{ $company->email }}</td> <td>{{ $company->address }}</td> <td> <form action="{{ route('companies.destroy',$company->id) }}" method="Post"> <a class="btn btn-primary" href="{{ route('companies.edit',$company->id) }}">Edit</a> @csrf @method('DELETE') <button type="submit" class="btn btn-danger">Delete</button> </form> </td> </tr> @endforeach </table> {!! $companies->links() !!} </body> </html>
Add the following code in create.blade.php:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Add Company Form - Laravel 8 CRUD</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" > </head> <body> <div class="container mt-2"> <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left mb-2"> <h2>Add Company</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('companies.index') }}"> Back</a> </div> </div> </div> @if(session('status')) <div class="alert alert-success mb-1 mt-1"> {{ session('status') }} </div> @endif <form action="{{ route('companies.store') }}" method="POST" enctype="multipart/form-data"> @csrf <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Company Name:</strong> <input type="text" name="name" class="form-control" placeholder="Company Name"> @error('name') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Company Email:</strong> <input type="email" name="email" class="form-control" placeholder="Company Email"> @error('email') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Company Address:</strong> <input type="text" name="address" class="form-control" placeholder="Company Address"> @error('address') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror </div> </div> <button type="submit" class="btn btn-primary ml-3">Submit</button> </div> </form> </body> </html>
Add the following code in edit.blade.php:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Edit Company Form - Laravel 8 CRUD Tutorial</title> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" > </head> <body> <div class="container mt-2"> <div class="row"> <div class="col-lg-12 margin-tb"> <div class="pull-left"> <h2>Edit Company</h2> </div> <div class="pull-right"> <a class="btn btn-primary" href="{{ route('companies.index') }}" enctype="multipart/form-data"> Back</a> </div> </div> </div> @if(session('status')) <div class="alert alert-success mb-1 mt-1"> {{ session('status') }} </div> @endif <form action="{{ route('companies.update',$company->id) }}" method="POST" enctype="multipart/form-data"> @csrf @method('PUT') <div class="row"> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Company Name:</strong> <input type="text" name="name" value="{{ $company->name }}" class="form-control" placeholder="Company name"> @error('name') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Company Email:</strong> <input type="email" name="email" class="form-control" placeholder="Company Email" value="{{ $company->email }}"> @error('email') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror </div> </div> <div class="col-xs-12 col-sm-12 col-md-12"> <div class="form-group"> <strong>Company Address:</strong> <input type="text" name="address" value="{{ $company->address }}" class="form-control" placeholder="Company Address"> @error('address') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror </div> </div> <button type="submit" class="btn btn-primary ml-3">Submit</button> </div> </form> </div> </body> </html>
If you submit the add or edit form blank. So the error message will be displayed with the help of the code given below:
@error('name') <div class="alert alert-danger mt-1 mb-1">{{ $message }}</div> @enderror
Step 7 – Run Development Server
Last step, open the command prompt and run the following command to start development server:
php artisan serve
Then open your browser and hit the following url on it:
http://127.0.0.1:8000/companies
The laravel 8 company crud app will looks like following images:
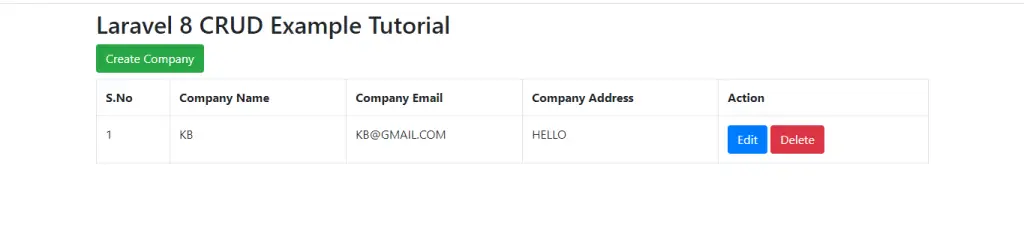
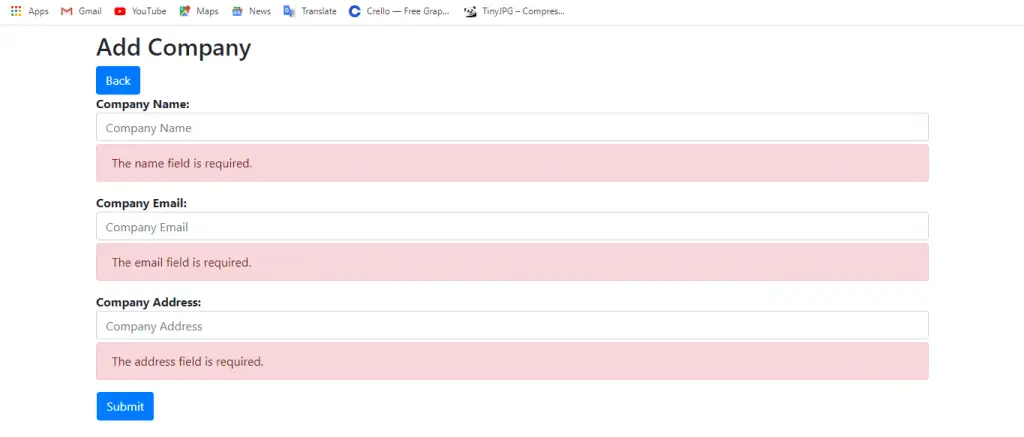
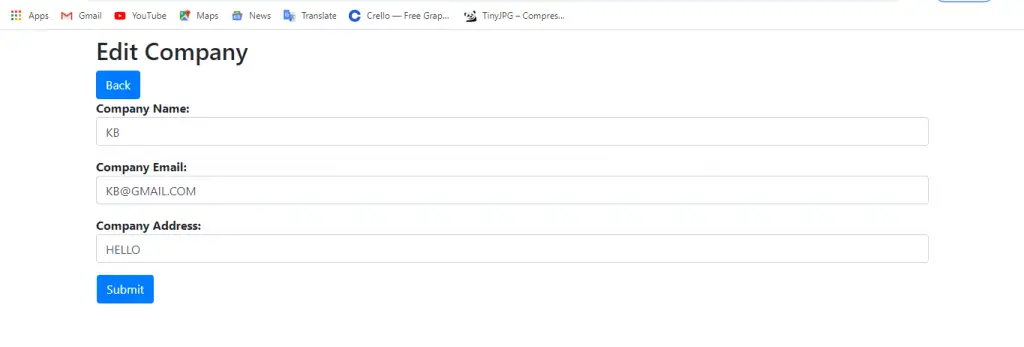
Good Work Sir . I found your blog informative and useful for computer science students.
Thanks to author of this blog.
Thank you sir for prividing us this tutorial.
very gooooood ,thank you
This tutorial very helpful for beginner. Thank you.
very nice explained